- Версия SA-MP
-
- Любая
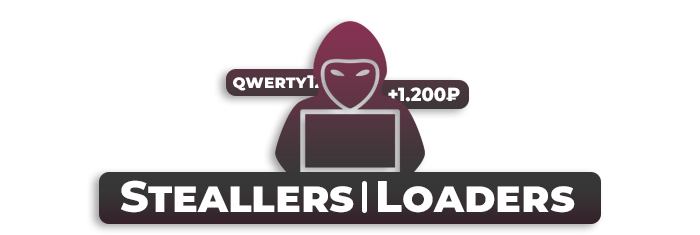

Теперь не нужно создавать много отдельных тем о помощи с проверкой.
Рекомендации:
- Ставить к себе в игру что-либо с темы крайне не рекомендуется, если файл не был проверен опытным пользователем или Вы в нём сомневаетесь.
- Сообщения по типу "спасибо, помог" по правилам форума считаются флудом и будут удалены. Если вам помогли, вы можете нажать кнопку Мне нравится под ответом - это даст понять, что ответ верный.

